How to create object -dialog- from library ?
-
OK, I have forgotten how to do it ... senior moment ...
I have build and added (external) C++ Linux library .
Using external because I followed the wizard and it worked ...
Will test internal later.
I have added a simple button dialog to the library and now like to test it in my main program.
Addi the library added the following to main project fileunix:!macx: LIBS += -L$$PWD/../../../SUB_LIBRARY/build-SUB_TEST_LIBRARY-Desktop_Qt_5_15_2_GCC_64bit-Debug/ -lSUB_TEST_LIBRARY INCLUDEPATH += $$PWD/../../../SUB_LIBRARY/build-SUB_TEST_LIBRARY-Desktop_Qt_5_15_2_GCC_64bit-Debug DEPENDPATH += $$PWD/../../../SUB_LIBRARY/build-SUB_TEST_LIBRARY-Desktop_Qt_5_15_2_GCC_64bit-Debug
This is my test library project
QT -= gui TEMPLATE = lib DEFINES += SUB_TEST_LIBRARY_LIBRARY CONFIG += c++11 # You can make your code fail to compile if it uses deprecated APIs. # In order to do so, uncomment the following line. #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 SOURCES += \ dialog.cpp \ sub_test_library.cpp HEADERS += \ SUB_TEST_LIBRARY_global.h \ dialog.h \ sub_test_library.h # Default rules for deployment. unix { target.path = /usr/lib } !isEmpty(target.path): INSTALLS += target FORMS += \ dialog.ui
I was hoping intelisense will help me to create dialog object using the library
-something likevoid Form_Widget_HCI::on_pushButton_65_clicked() { qDebug() << "test library ...."; ui->lineEdit_9->setText("on_pushButton_65_clicked"); ui->lineEdit_9->setText("test library"); // create test dialog SUB_TEST_LIBRARY *pointer = new SUB_TEST_LIBRARY(); }
My question is
what is correct syntax to create the library dialog?
It would help me if somebody could describe the sequence of events leading to "new" code. How both projects files and various includes interact.
Please try NOT to refer TOO much to make..,. -
I'll rephrase my post AFTER reading this
https://wiki.qt.io/How_to_create_a_library_with_Qt_and_use_it_in_an_application
-
It seems that writers of the tutorials cannot agree on terminology.
Why do they stress "shared" library ? Superficial / duplicate designation...
It looks as Windoze uses "dynamic loaded library" - DLL
and Linux is fond of "static" library.Making progress . but
I am still looking for better explanation of "linking / dependencies " details...
(maybe there is a pony there somewhere ...) -
Hi,
.dll files are shared libraries.
@AnneRanch said in How to create object -dialog- from library ?:
and Linux is fond of "static" library.
That's wrong. Take a look at your Linux applications, they are all using shared libraries.
As for your original question, you should use it in the same manner as any other libraries. There's really not much more to it than that.
Add the path to you library headers to the INCLUDEPATH of the projects where you want to use it and add the path and library name to the LIBS variable.
-
I am sorry, but I missed your last reply.
So this is duplicate post AND still need a solution.
Where is the header file?
Was it added by wizards ??If the library header DOES not exists compiler usually complains .
My code builds - no problem.Still missing "header" reference in main project.
After following almost 30 steps in coding main project and adding test library
I am sill missing "header" reference in main project.Normally - main project should have included library header file..
Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += targetunix:!macx: LIBS += -L$$PWD/../build-TestLibraryProject-Desktop_Qt_5_15_2_GCC_64bit-Debug/ -lTestLibraryProject
INCLUDEPATH += $$PWD/../build-TestLibraryProject-Desktop_Qt_5_15_2_GCC_64bit-Debug
DEPENDPATH += $$PWD/../build-TestLibraryProject-Desktop_Qt_5_15_2_GCC_64bit-Debug -
INCLUDEPATH should point to the folder(s) where you library headers reside.
-
@SGaist I think I found the "problem```
The library is L/l "linked" correctly, but in separate folder, not in same as the project. I do nor recall specifying such folder ...However the INCLUDEPATH is just that - include PATH only .
Looks as the library HEADER has to be added manually
WHERE and HOW?Did I missed part of the wizard ?
I did this years ago I do not recall how I solved it - my old project is long gone.
I keep better log nowunix:!macx: LIBS += -L$$PWD/../build-TestLibraryProject-Desktop_Qt_5_15_2_GCC_64bit-Debug/ -lTestLibraryProject
INCLUDEPATH += $$PWD/../TestLibraryProject
DEPENDPATH += $$PWD/../TestLibraryProject -
No, there should be no need to add any header. Your custom library should be used like any other library that resides in a non system path.
-
Help me to understand or disagree
-
I am adding external library
I am selecting : -
Library type - as selected - hence Linux
-
(Actual ) Library file ...so - links fine
-
(Actual ) Library path - as above
-
Nowhere in this step / dialog there is a reference to "include " header or no actual PATH to the library source / header
-
There is not or I just cannot see it , a reference tying main and library projects .pro files together.
-
-
OK, I am not sure this is correct approach, but so far it works - main app builds
test dialogs from two "shared" libraries.- Create real "sub project"
- Add main app project to it as new main sub project
- Create / add Linux library as add new sub project
- Add newly created library to main app (sub project) - as an internal (!) library - using defaults.
- ADD library include headers to main sub project (!)
- rebuild "sub project" , run and watch for smoke...
#include "mainwindow.h" #include "ui_mainwindow.h" // add library includes #include "SUB_LIBRARY_1_global.h" #include "testdialog.h" #include "sub_library_1.h" #include "SUB_LIBRARY_2_global.h" #include "testdialog_1.h" #include "sub_library_2.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent) , ui(new Ui::MainWindow) { ui->setupUi(this); TESTDialog *TD = new TESTDialog(); TD->show(); TESTDialog_1 *TD_1 = new TESTDialog_1(); TD_1->show(); } MainWindow::~MainWindow() { delete ui; }
-
You should only include the header of the class you use. There should be no need to include the XXX_global.h which I guess contains the export macros and are is already included in the header of the classes you wrote in your libraries.
-
@SGaist I have cheated a little . When I did this exercise with my previous project I did checked include guards ., Not this time , but I do not have multiple includes anyway... Just being lazy or let the code do its work...
However, I am not totally out of the woods...,
Using "sub-project" to manage main and library so far only works when I add " new sub project- library. " and then add newly created library to main project.
(I realize these terms sound stupid but I am no going to invent my own terminology - this is a TWO step process- create and add library as sub project
- add / link such library to main project
So far I am back to very original question - doing the above with EXISTING project / library does not function.
The existing code is NOT in sub project tree...
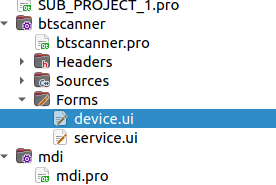 code_text
Here is btscanner in my SUB_PROJECT_1
Here is my device.ui form in QTDesigner
..and here is my ":run time" dialog
obviously NOT the same form as in SUB_PROJECT_1
PS I do not expect much help from the group
on this one. Hope it is "back" to inlcudes... -
The 'Include path' line in the dialog should be set to the folder where the header files (*.h) of the library are located and not the build folder.
In regards to it not asking for the location of a .pro file, this is for adding any external library, so it does not have to be a Qt-based library built using a .pro file. Also, an external library in this case refers to a pre-built library (the .so/.a/.dll/.lib file already exists) and won't be compiled as part of your project so there is no need for your project to know about the library's .pro file.
-
Appreciate the comments however it does no explaining the relations between subproj and its projects. I still would like for somebody to confirm or dispute the way I am coding my project. I am avoiding terms build or create here.
I start with coding TEST_SUBDIR_PROJECT
New File ->Other project -subdir.
the result is ability to add (first) executable subproject.
I usually start with add of QT application - widget.
After that I "build " and "run" the application.
There are no includes / external libraries / internal libraries - all standard QT application .Then I add new TEST C++ library as a independent project
Then I add TEST C++ library as new subproject to TEST_SUBDIR_PROJECT
That is another sub project managed by subdirs. BUT it is now an internal library too. Not useful at this point.
BUT it can be added as library to both TEST_SUBDIR_PROJECT ,pro and MAIN .pro files.
I have not figured out how TEST_SUBDIR_PROJECT .pro can use it , but it allows MAIN .pro file to use the added TEST library - and that is the entire purpose of this excise....NOW I can add required includes to main.cpp and ACTUALLY USE the library.
THE (hopefully) LAST and repeated question remains - what are all these includes in
TEST C++ library FOR?QT -= gui widgets dialog QT += widgets #added widgts TEMPLATE = lib DEFINES += TEST_LIBRARY_LIBRARY CONFIG += c++11 # You can make your code fail to compile if it uses deprecated APIs. # In order to do so, uncomment the following line. #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 SOURCES += \ test_library.cpp \ testdialog.cpp HEADERS += \ TEST_LIBRARY_global.h \ test_library.h \ testdialog.h # Default rules for deployment. unix { target.path = /usr/lib } !isEmpty(target.path): INSTALLS += target FORMS += \ testdialog.ui
Next step is to convert existing .pro (QT excising example brscanner) to library , add it as library subproject and then add it as another library (now internal) to the main project.
-
Could somebody kindly help me to figure out LAST part of my LAST post ?
Since I am a lazy person I like to reuse somebody else code.
I have made wrong choice and reused "btscanner" example BUT I modified it and interwoven it into my code. Now I regret this.
I am now using the same code as a library and have modified my main code as such. It works with ONE exception - I need to remove all of the interwoven code .
Part of the removal is to REMOVE references added to my .pro files - including "includes ". ( see mine previous post )I prefer NOT to remove these references without some knowledge what is their purpose.
Personally since I am now able to modify the library code I would prefer other ways to discard the interwoven code - perhaps renaming the files...