Copying the content of int array to QString
-
Hi Friends
Help need to convert integer array To QString and emit the data to my slot
int a[10]={196,192,200,194,178,147,196,200,194,200};
I already tried this
char text[] = { '1', '2', '3', '4', '5', '\0' }; QString string(text); qDebug()<<string; emit Send_Data_Array_to_form(string);
above snippet work perfectly. But when i send integer array to QString the Receiver slot not properly receive my data i don know why. help me to resolve this issue.
this is my receiver slot
void MainWindow::Test_Array_Initialization(QString S_Data) { qDebug()<<"*****SIGNAL EMITED From My_signal_get_form1**********"<<endl; qDebug()<<"data received"<<S_Data.size()<<S_Data; }
Thanks in Advance.
-
Please post the code which gets the int array and sends it. It is the crucial part of the code in this case.
Please also clarify what exact output do you expect (what S_Data you think should look like).
-
hi@sierdzio I have problem with convert int array to QString . I just compiled my own test code
void Array_Data_emit_Form1::Raw_Data_Initialization() { unsigned int a[5]={1,2,3,-4,5}; QString My_String; for(int i=0; i<5; i++) { My_String=a[i]; } qDebug()<<"My_String datas:"<<My_String; emit SEND_Array_To_Mainw(My_String); }
This is my connect signal in mainwindow.cpp
```
connect(data_connect,SIGNAL(SEND_Array_To_Mainw(QString)),this,SLOT(Show_Data_to_Window(QString)));
This is my Receiver Slot in mainwindow.cpp
void MainWindow::Show_Data_to_Window(QString S_data)
{qDebug() <<"Data Received"<<S_data.size()<<S_data;
}
The Out put I saw in Console My_String datas: "" Data Received 1 "" My_String datas: "" Data Received 1 ""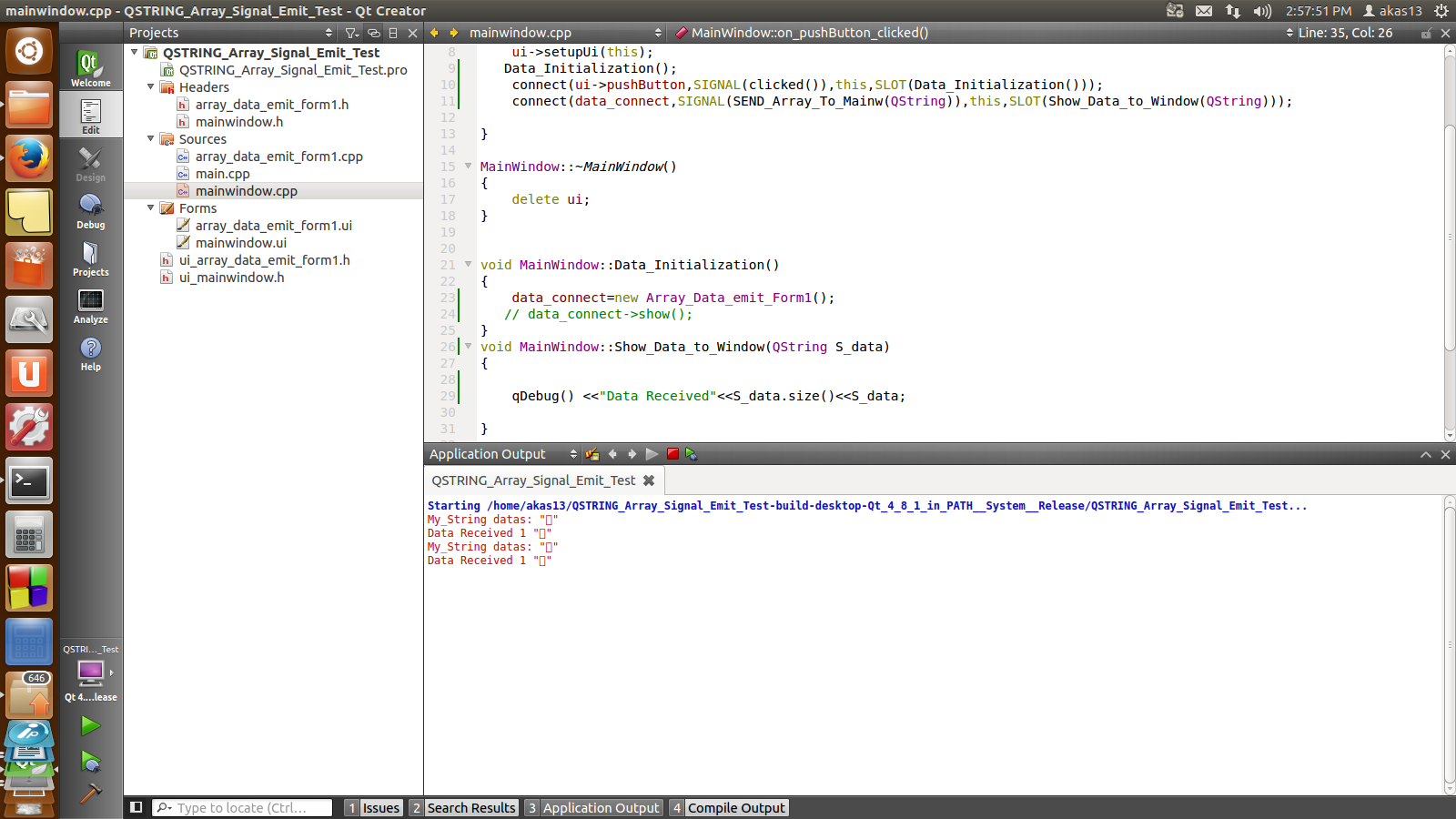 What I expect is My array data will convert to String In Receiver slot the array data must be converted to string like 1 2 3 -4 5 Help me to resolve this issue Thanks in advance
-
@vivekyuvan said in Copying the content of int array to QString:
unsigned int a[5]={1,2,3,-4,5};
QString My_String;
for(int i=0; i<5; i++)
{
My_String=a[i];
}You replace My_String each time in this loop. You probably want something like this instead:
My_String+=QString::number(a[i]);
-
@vivekyuvan what you need is to convert the raw int to a QStirng. Lucky for you QString has a method that does that for you
do the following change:
QString My_String; for(int i=0; i<5; i++) { My_String.append(QString::number(a[i])); }
and you should be fine.
Edit: @sierdzio was a bit faster x) but great minds think alike *cough*
-
hi@sierdzio and @J-Hilk Thanks for the reply. I tried both method but no luck
this is what my output in console window.
My_String datas: "12342949672925" Data Received 14 "12342949672925"
here is the change in my Sending slot:
void Array_Data_emit_Form1::Raw_Data_Initialization() { unsigned int a[5]={1,2,3,-4,5}; QString My_String; for(int i=0; i<5; i++) { //My_String=a[i]; My_String+=QString::number(a[i]) //My_String.append(QString::number(a[i])); } qDebug()<<"My_String datas:"<<My_String; emit SEND_Array_To_Mainw(My_String); }
-
If you want spaces between the numbers... insert them :-)
My_String+=QString::number(a[i]) + " ";
Also, another issue:
unsigned int a[5]={1,2,3,-4,5};
"unsigned" type will not store -4. It's actually quite surprising that the compiler does not throw an error here.
-
@sierdzio thank you. Sorry for the silly mistake that made by me. I just changed datatype as signed integer now its working i got 123-45. now my problem solved.
```
signed int a[5]={1,2,3,-4,5};
-
@vivekyuvan it is doing exactly what yopu tell it to,
unsigned int can be anything between 0 and 4,294,967,295 : -4 therefore => 4,294,967,292 -
@sierdzio said in Copying the content of int array to QString:
It's actually quite surprising that the compiler does not throw an error here.
There's no error from the compiler's point of view, at best it should be a warning. There's no significant difference between signed and unsigned numbers aside from our interpretation of the data, so the compiler has no reason to complain. If you consider this:
unsigned short x = -3; // 0xFFFD unsigned short y = 5; // 0x0005 short z = x + y; // = 0xFFFD + 0x0005 = 0x10002 -> (due to truncation from overflow) 0x0002 == 2 short z2 = x - y; // = 0xFFFD - 0x0005 = 0xFFFD + 0xFFFB = 0x1FFF8 -> (due to truncation from overflow) 0xFFF8 == -8
It's perfectly valid from the compiler's point of view, it's just a hell of a confusing way to do things and can get you in big trouble whenever comparing is involved. ;)
-
Yes, thanks for the explanation.
My intuition here was that since we clearly and openly want unsigned, the compiler will be clever enough to point out that "-4" is likely a bug. With gcc and clang being pretty clever about a lot of things (like pointing out signed/ unsigned mismatches in if statements etc.) I assumed it would shoot some (at least) warning here.
Actually, maybe it did print a warning but OP ignored it, who knows.
-
It may not be a bug, that's what one could use, albeit there are better ways, to get the upper limit of the unsigned type.
unsigned maxint = -1; // And voila we have the UINT_MAX
Although, I agree that perhaps a warning is in order, this doesn't seem to be the case with g++ (7.2.x) on my machine. The above line will not generate even a warning and I do compile with
-Wall
.like pointing out signed/ unsigned mismatches in if statements etc.
This is when it comes to comparisons, because due to representation (and integral promotion)
-1
is the biggest number there possibly can be in a mixed signed-unsigned comparison.Actually, maybe it did print a warning but OP ignored it, who knows.
It'd depend on the actual compiler, but it might not have warned him.